I was helping my wife to setup a Facebook page for her business Adore Premium . I needed to delete a lot of posts.
It came to my attention that there is no way to select multiple posts and delete them the in Meta Business Suite.
So I decided to write a script to select multiple posts. Then I could delete them in bulk.
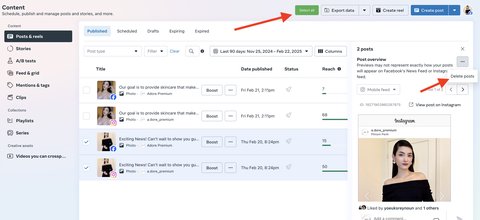
Show me the code
This is a UserScript
. I use
Violentmonkey
to inject this script into Meta Business Suite.
// ==UserScript==
// @name Select all posts & reels
// @namespace Violentmonkey Scripts
// @match *://business.facebook.com/*
// @require https://cdn.jsdelivr.net/npm/@violentmonkey/dom@2
// @grant none
// @version 1.0
// @author -
// @description 2/19/2025, 5:30:35 PM
// ==/UserScript==
const disconnect = VM.observe(document.body, () => {
// Find the target node
const node = document.querySelector('#mediaManagerExportInsightsButton');
var count = 0;
if (node) {
const btn = document.createElement('button');
btn.textContent = 'Select all';
btn.style.backgroundColor = '#4CAF50';
btn.style.color = 'white';
btn.style.padding = '10px 15px';
btn.style.border = 'none';
btn.style.borderRadius = '4px';
btn.style.cursor = 'pointer';
btn.addEventListener('click', async function() {
const checkboxes = document.querySelectorAll('td input[aria-checked="false"][type="checkbox"]');
const checkboxArray = Array.from(checkboxes);
const end = Math.max(0, checkboxArray.length - 20);
for (let i = checkboxArray.length - 1; i >= end; i--) {
const checkbox = checkboxArray[i];
// Random delay between 500ms and 2500ms (0.5 to 1 seconds)
const randomDelay = Math.floor(Math.random() * 500) + 500;
// Wait for the random delay
await new Promise(resolve => setTimeout(resolve, randomDelay));
// Small additional random delay before actual click (100-300ms)
await new Promise(resolve => setTimeout(resolve, Math.random() * 200 + 100));
if (i % 5 === 0) {
// Scroll element into view before clicking (more human-like)
checkbox.scrollIntoView({ behavior: 'smooth', block: 'center' });
// Wait a bit after scrolling
await new Promise(resolve => setTimeout(resolve, Math.random() * 300 + 200));
}
// Finally click the checkbox
checkbox.click();
count++;
// Log for debugging (optional)
console.log(`Clicked checkbox after ${randomDelay}ms delay`);
}
btn.textContent = 'Select all (' + count + ')';
// checkboxes.forEach(checkbox => checkbox.click());
alert("selected " + checkboxes.length);
});
node.parentElement.parentElement.parentElement.parentElement.prepend(btn);
// disconnect observer
return true;
}
});
How does the script work?
Here’s an explanation of what this script does:
- It targets the Facebook Business Manager page ( https://business.facebook.com/) .
- The script adds a new “Select all” button next to the “Export Insights” button on the page.
- When clicked, this button selects multiple posts by clicking their checkboxes.
Key features:
- It selects up to the last 20 unselected items (checkboxes).
- It introduces random delays between selections to mimic human behavior.
- It scrolls to make checkboxes visible before clicking them.
- It updates the button text to show how many items were selected.
Is it safe?
While I can’t guarantee absolute safety, I can provide some insights on potential risks and considerations:
- Facebook might detect and flag automated behavior, potentially leading to account restrictions.
Rapid actions could be mistaken for bot activity
.
- The script includes delays to mimic human behavior, which helps, but may not be foolproof.
- Facebook could change their UI, breaking the script’s functionality.
- Selecting many items quickly might strain browser performance.
- The script doesn’t access sensitive data, but always be cautious with third-party scripts.